Reading From Standard In
In C++, we can read in a single variable the way we learned in class:
#include <iostream> int main() { int myVar; cout << "Please enter a variable:"; cin >> myVar; }
This will read from standard in and store the result into cout. The program will stop reading once it hits whitespace.
We can also read an entire line with the "getline()" command. The "getline()" method takes two arguments--the variable we are going to store into, and the length of the string.
#include <iostream> using namespace std; #define BUF_SIZE 80 int main(int argc, char **argv) { char line[BUF_SIZE]; cout << "Now, enter a name: "; cin.getline(line, BUF_SIZE); cout << "Your name is " << line; return 0; }
Input Gotcha
When mixing getline() and other input methods, there are a few things to consider. getline() reads and discards the ending '\n' character, but the other input methods do not--they stop just before it. They do skip over '\n' as leading whitespace, though. This can lead to unexpected results. For example:#include <iostream> using namespace std; int main(int argc) { int i1; char s1[BUF_SIZE]; char s2[BUF_SIZE]; cin >> i1; cin.getline(s1, BUF_SIZE); cin.getline(s2, BUF_SIZE); return 0; }
If we input:
2 Hello World
The program will exit immediately after we input Hello. i1 will contain '2', but s1 will contain an empty string (it will read the rest of the line, which will contain only a newline character, which is subsequently stripped off by getline()), and s2 will contain 'Hello'. To avoid this, an extra getline() can be inserted after reading in the int with "cin >> i1" to discard the '\n'. Here is a visual representation of what is happening on the input buffer:
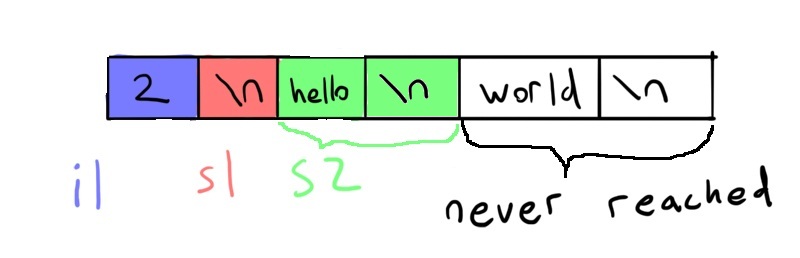