Copy Constructor in Rectangle Class
A copy constructor is a constructor with a single argument of the same type as the class. Making a copy of an object requires a special method called a copy constructor. The copy constructor should create an object that is a separate, independent object, but with the instance variables set so that it is an exact copy of the argument object.
You will create a copy constructor in the Rectangle
class. Because of composition, you will have to create a copy constructor in
the Point class and use it in the Rectangle class. Note that Point is
immutable, so that the copy constructor in the Point class is not really
necessary.
// copy constructor in Point class public Point(Point original) { // Add your code here } // copy constructor in Rectangle class, which // will use the copy constructor in Point class public Rectangle(Rectangle original) { upperLeft = new Point(original.upperLeft) // Finish the rest of the code for another // three instance variables }Note that the following copy constructor is COMPLETELY WRONG:
public Rectangle(Rectangle original) { upperLeft = original.upperLeft; }This is called aliasing. What this WRONG constructor is doing is setting the pointer to upperLeft equal tpoint to the original Rectangle's upper left, like so:
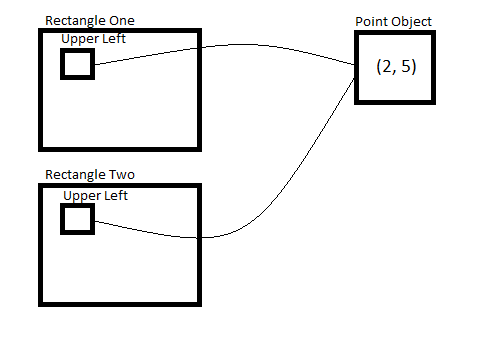
With this WRONG constructor, every time original's upper left changes, so wilour new object's upper left will change as well.
Testing
Uncomment the last part of the test program in main. Use the same test case as in step 6 to test your program.
Scanner scanner = new Scanner(System.in); int x = 1; int y = 1; System.out.print("Enter the x-coordinate of the upperLeft :"); x = scanner.nextInt(); System.out.print("Enter the y-coordinate of the upperLeft :"); y = scanner.nextInt(); Point upperLeft = new Point(x, y); System.out.print("Enter the x-coordinate of the lowerLeft :"); x = scanner.nextInt(); System.out.print("Enter the y-coordinate of the lowerLeft :"); y = scanner.nextInt(); Point lowerLeft = new Point(x, y); System.out.print("Enter the x-coordinate of the lowerRight :"); x = scanner.nextInt(); System.out.print("Enter the y-coordinate of the lowerRight :"); y = scanner.nextInt(); Point lowerRight = new Point(x, y); System.out.print("Enter the x-coordinate of the upperRight:"); x = scanner.nextInt(); System.out.print("Enter the y-coordinate of the upperRight:"); y = scanner.nextInt(); Point upperRight = new Point(x, y); Rectangle rectangle = new Rectangle(upperLeft, lowerLeft, lowerRight, upperRight); System.out.printf("Length of rectangle : %.2f\n", rectangle.getLength()); System.out.printf("Width of rectangle : %.2f\n", rectangle.getWidth()); System.out.printf("Area of rectangle : %.2f\n", rectangle.getArea()); System.out.printf("Perimeter of rectangle : %.2f\n", rectangle.getPerimeter()); //test the copy constructor Rectangle rectangleNew = new Rectangle(rectangle); System.out.printf("Length of rectangleNew : %.2f\n", rectangleNew.getLength()); System.out.printf("Width of rectangleNew : %.2f\n", rectangleNew.getWidth()); System.out.printf("Area of rectangleNew : %.2f\n", rectangleNew.getArea()); System.out.printf("Perimeter of rectangleNew : %.2f\n", rectangleNew.getPerimeter());